Unity Game Engine — Communicating with Javascript (Javascript Plugin)
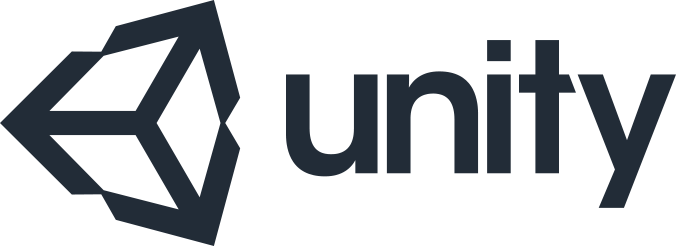
In this tutorial, I’ll show you how to send messages from Unity to Javascript. I’ll also show you how to send messages back from Javascript to your game. Sometimes this is also commonly referred to as creating Unity WebGL Plugins.
Setup
First, we’ll create a blank Unity Game. Note that you don’t have to use a blank template. I am doing this for simplicity. In Unity Hub, click “New Project”. Next, click Core on the right and choose a core project. I’m choosing the 2D core project. Give your project a name and click “Create Project”. Wait for your project to open automatically.
Switch Platform to WebGL
When Unity finally opens up with your blank project, it will look like this.
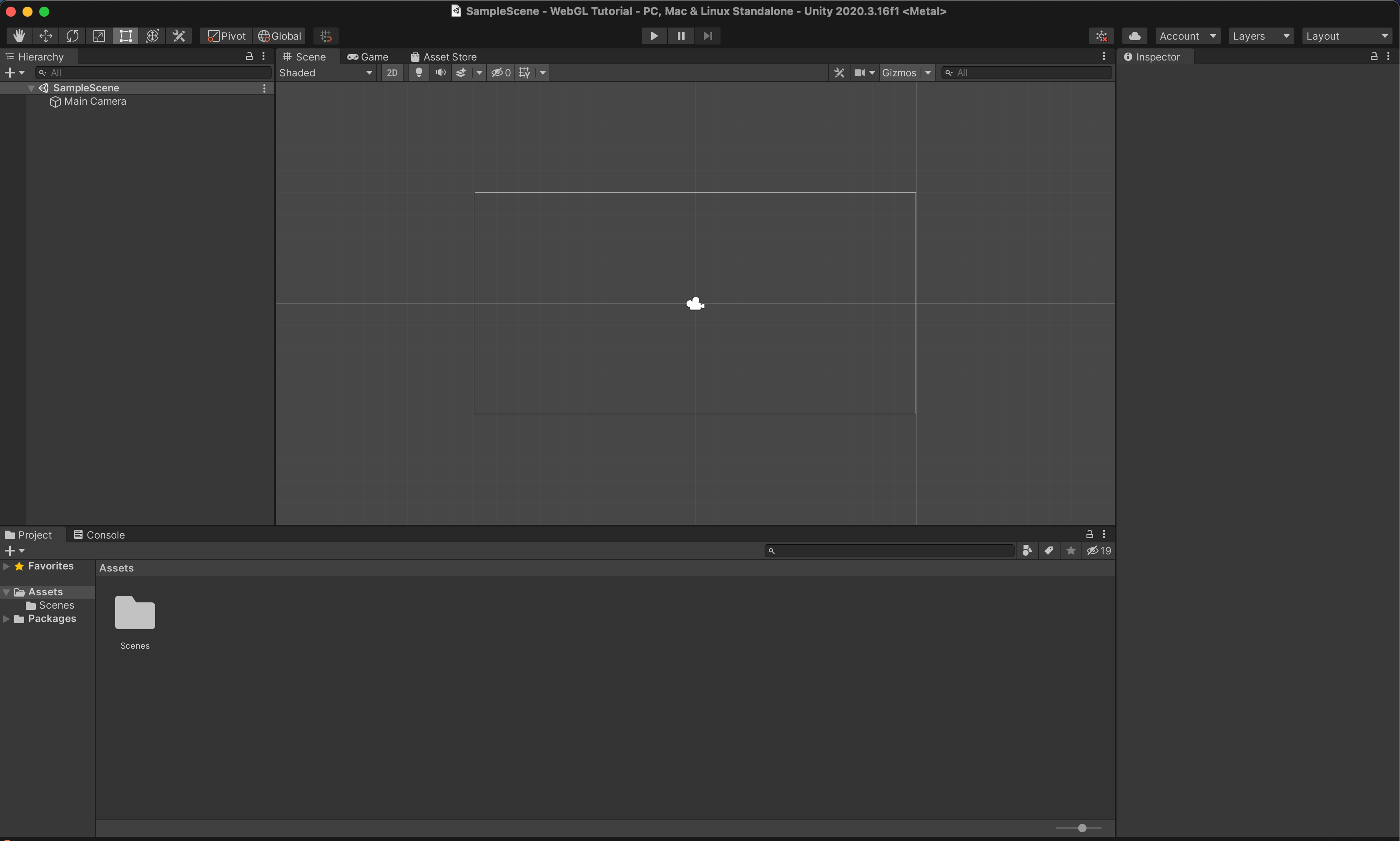
You will need to switch the platform to WebGL. While in Unity click File > Build Settings.
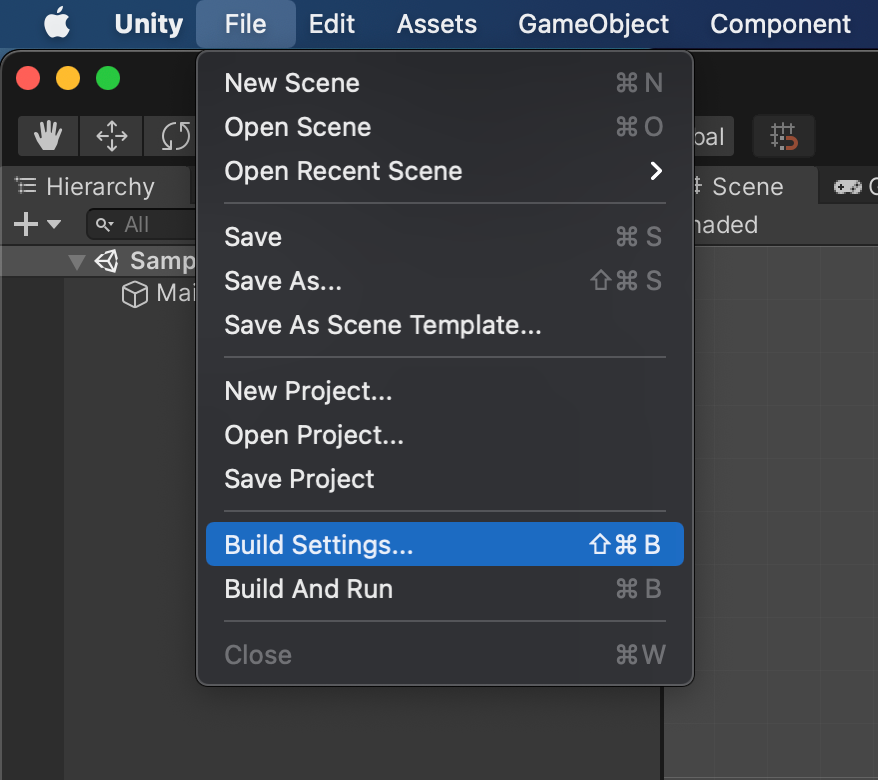
Next click on WebGL. You will then need to click on Switch Platform.
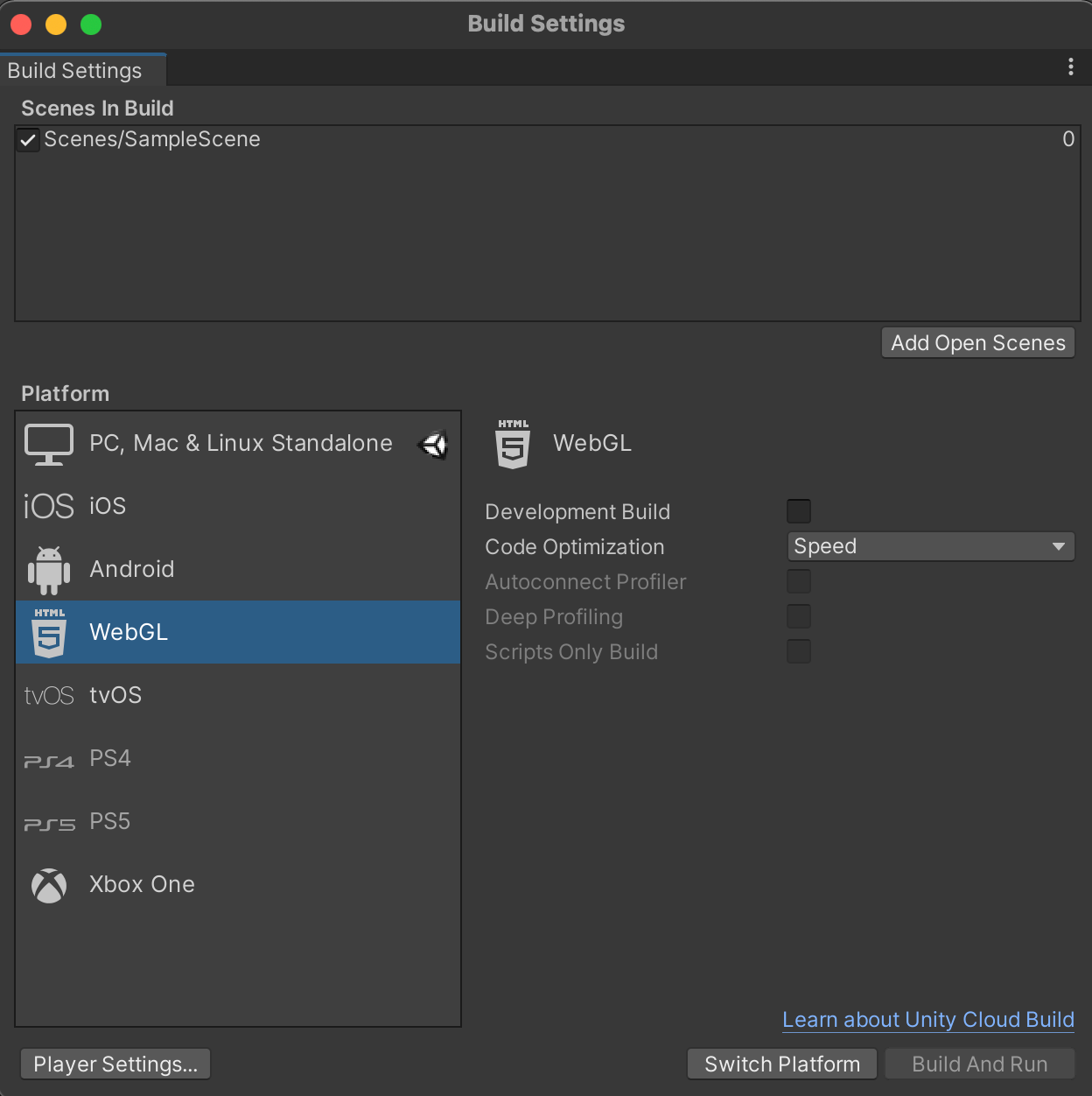
You know it has finally been switched when the Unity Logo is next to WebGL. “Build” and “Build and Run” buttons will also be present. Check the screenshot below.

You can now close the Build Settings Dialog/Pop-up.
Add a GameObject to your scene
Next, we can add a blank GameObject to your scene. Right-click on Sample Scene then click GameObject > Create Empty. This can be any GameObject. We are using a blank one for simplicity.
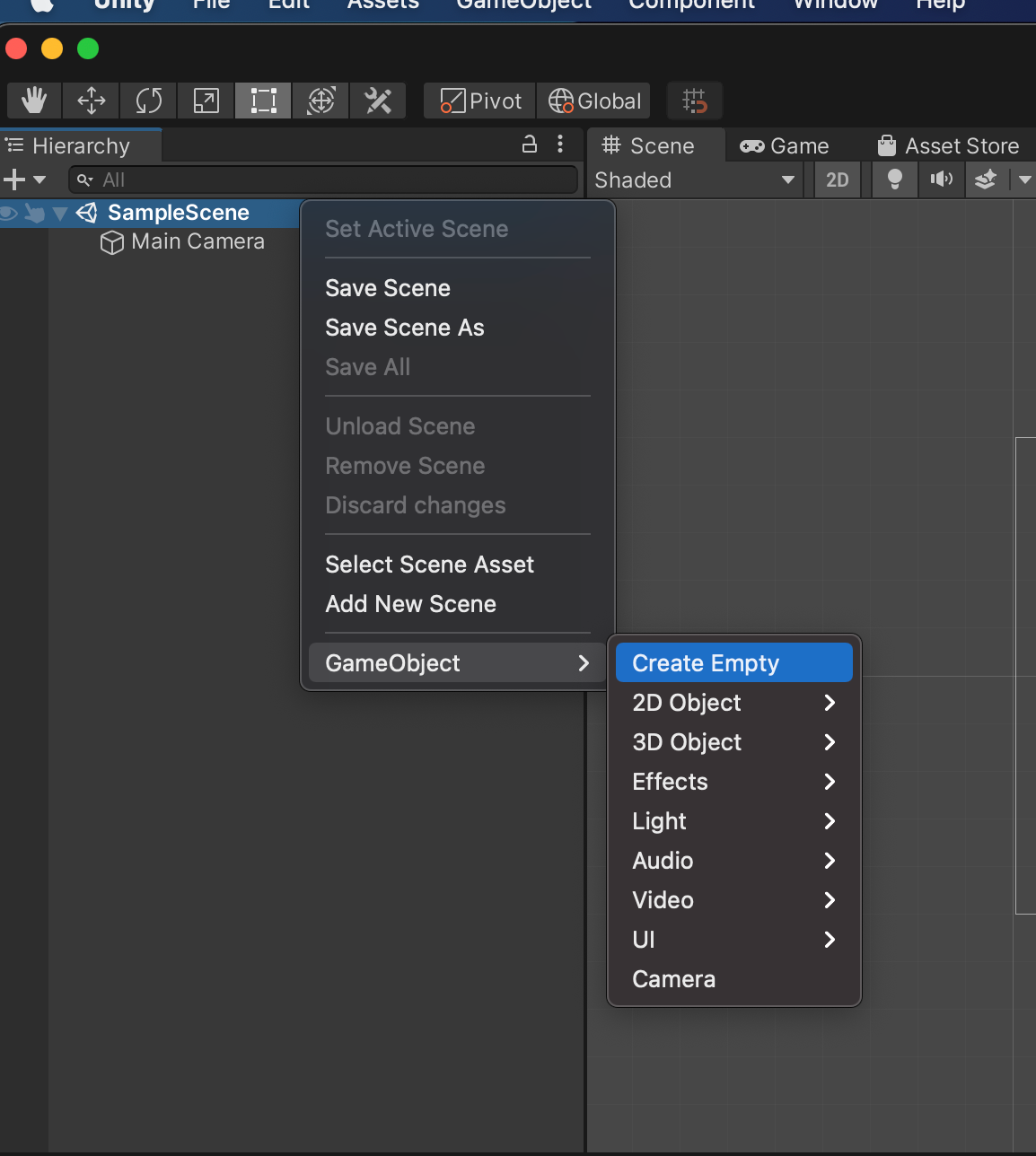
Next, rename the GameObject to ExampleBridge by Right-clicking on the GameObject > Rename. You can name this whatever you want, but it’s referenced when sending a message from Javascript to Unity, which we will cover soon.
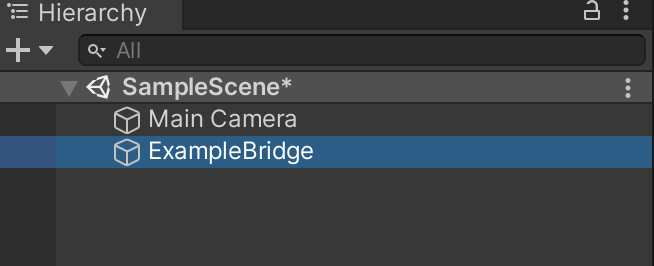
Now let’s create a new script. First, make sure to click on ExampleBridge and go to the Inspector tab which should be on the far right ( If you don’t see the inspector tab, in Unity you can click Window > General > Inspector ).
Click Add Component and search for “New Script”.
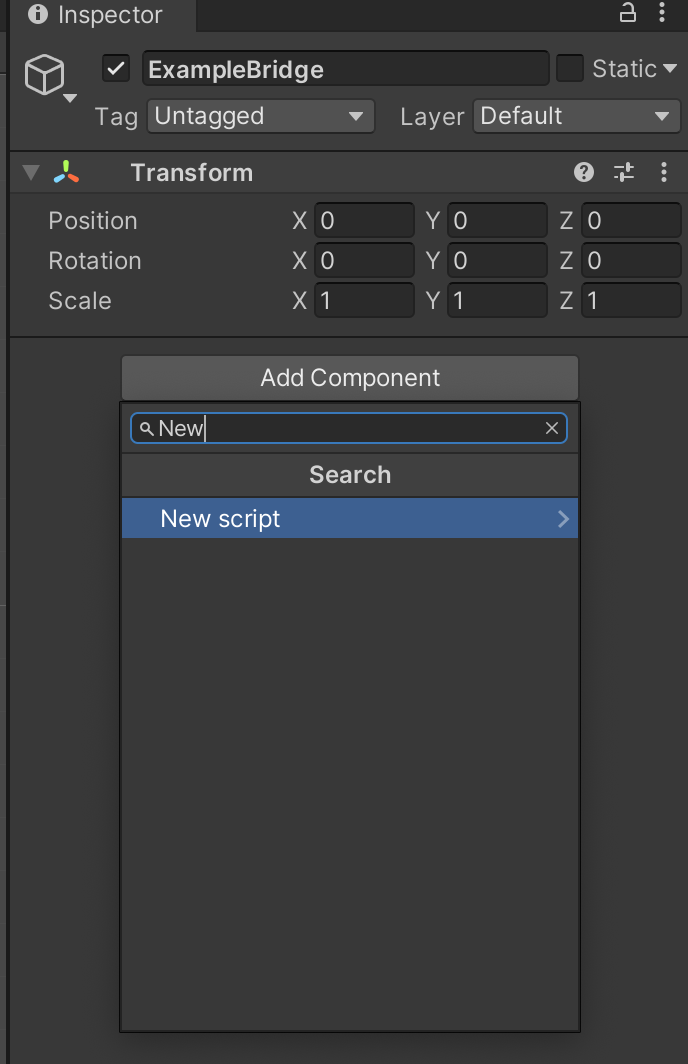
Click on New Script in the dropdown and name it ExampleBridge. Click Enter after typing the name to save it. You can also click the “Create and Add” button.
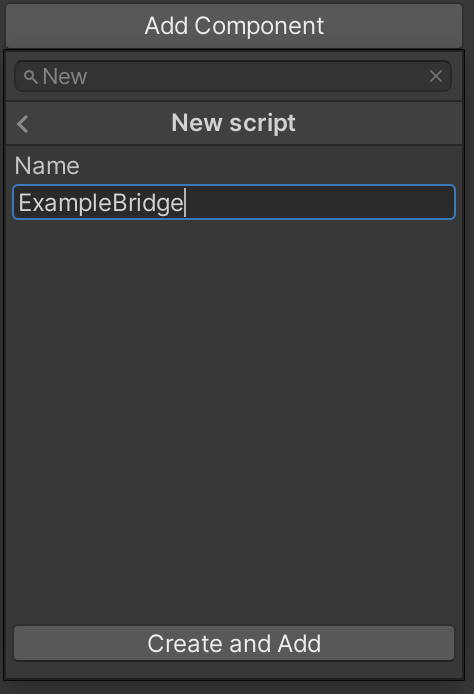
Create a Unity Javascript Plugin
Next, we will create a Unity Javascript Plugin to send messages to Javascript from Unity.
First, we will need to open the project in a code editor. I will use VS Code for this part.
You will notice there is an Assets folder. Create a folder under that named “Plugins”.
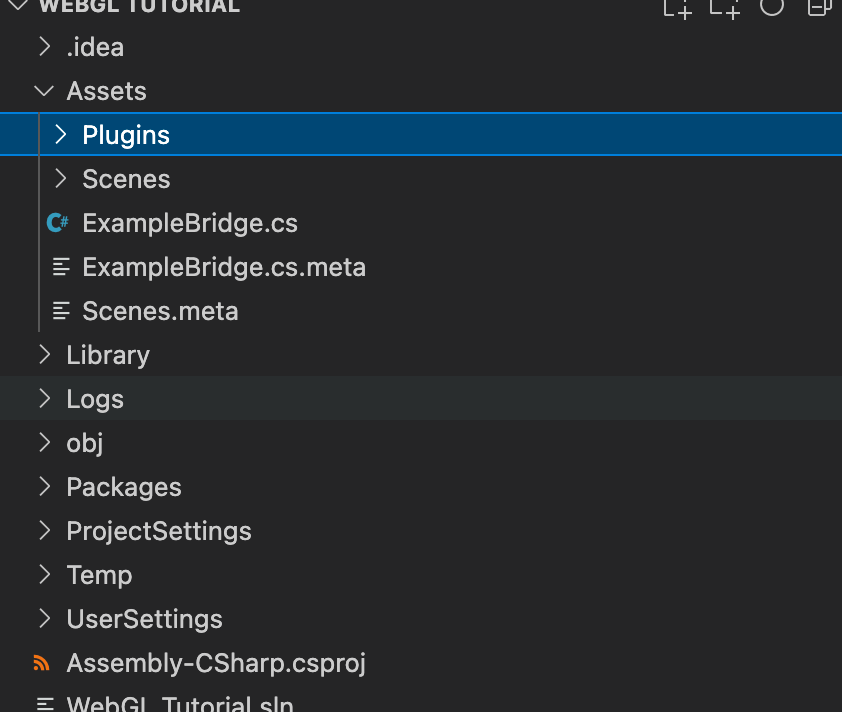
Next, create a file under that named ExamplePlugin.jslib
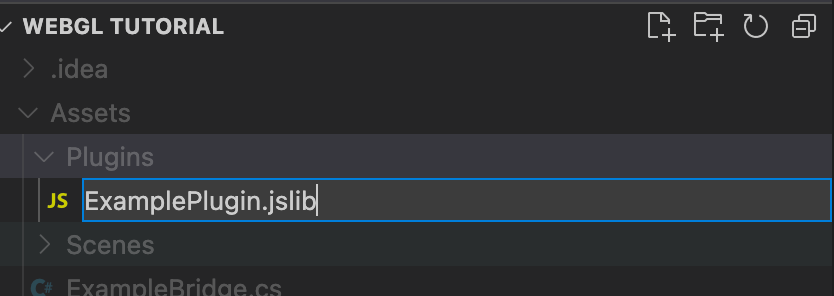
Add this code in ExamplePlugin.jslib
mergeInto(LibraryManager.library, {
HelloWorld: function (message) {
window.alert(message);
},
});
The main thing to focus on is the HelloWorld block of code. It’s a function called HelloWorld that accepts a parameter. In the curly braces, you can write any Javascript code you want. Here we are just showing an alert.
Next, open the ExampleBridge.cs script we created earlier. We are going to send a message to Javascript from this script. We need to add this code above the Start() method to let this script know about the plugin Javascript function.
[DllImport("__Internal")]
private static extern void HelloWorld(string message);
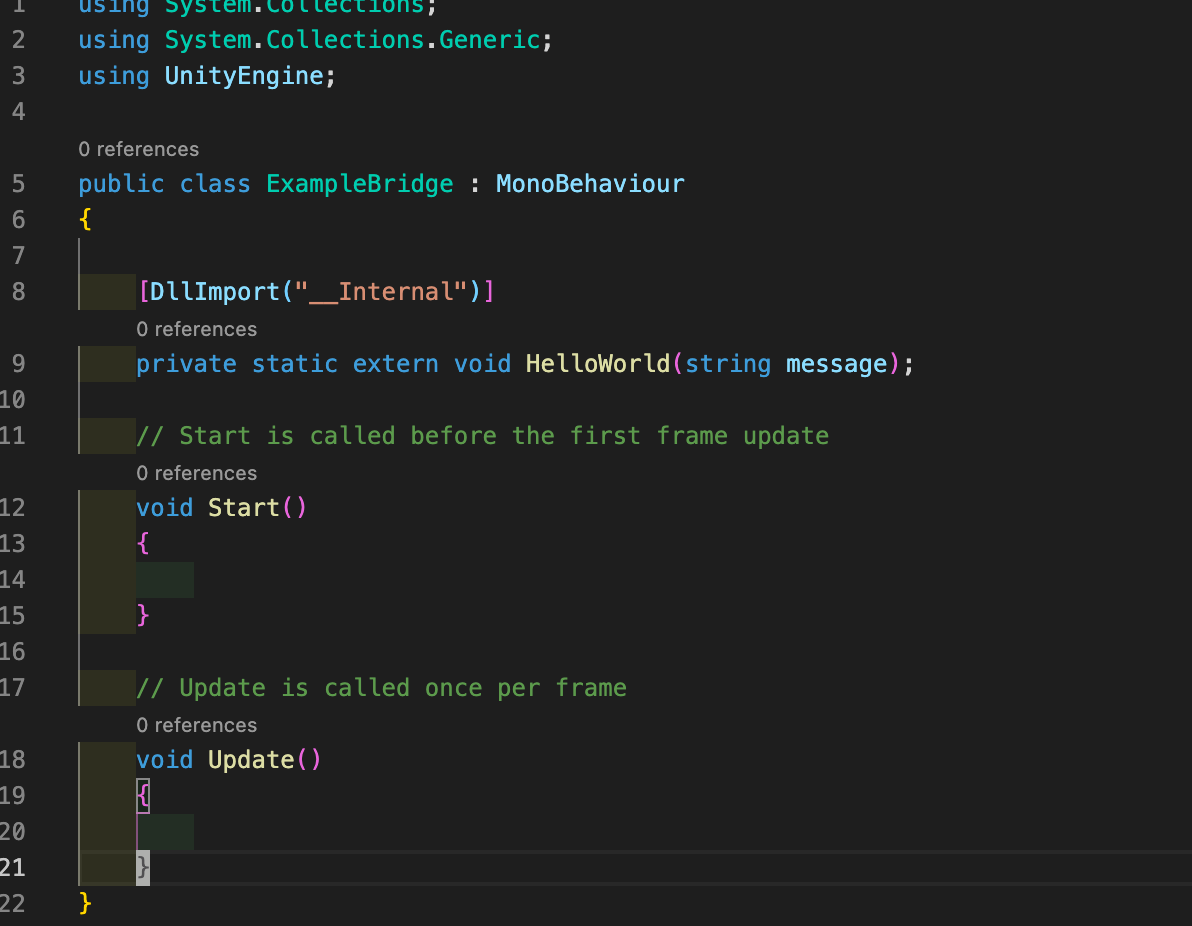
You also need to add this import:
using System.Runtime.InteropServices;
Add the code below to the Start method to call the Javascript function.
HelloWorld("Hello World");
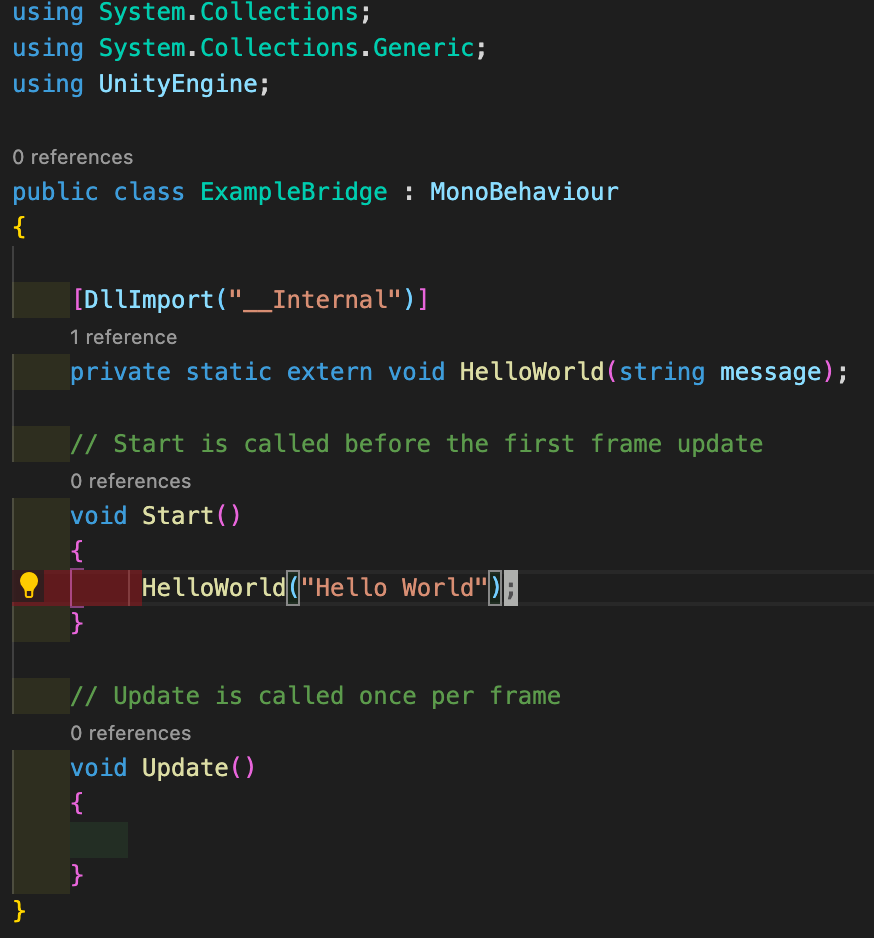
Let’s also add another method to get data back from Javascript. Add the code below. This will just print out a message, but it can be much more complex.
void MessageFromJavascript(string message)
{
Debug.Log(message);
}
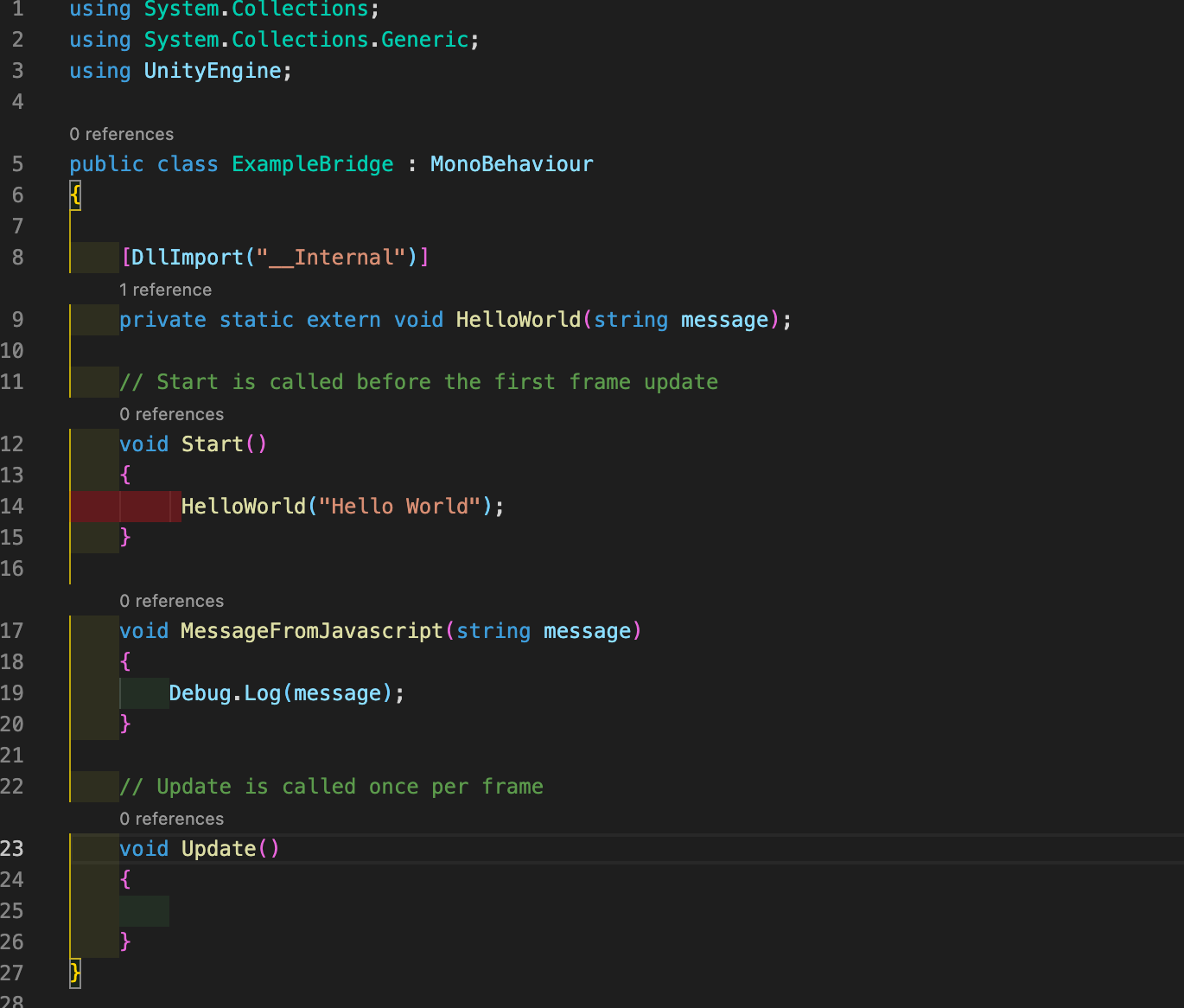
Now open up Build Settings in Unity again ( File > Build Settings ). Next click Build. It will ask what you want to save it as. We can name it unityWebTutorial for now. We need to build the game to write the code to send a message back from Javascript to Unity.
Send a message from Javascript to Unity
After Unity builds you will get a folder that looks like this.
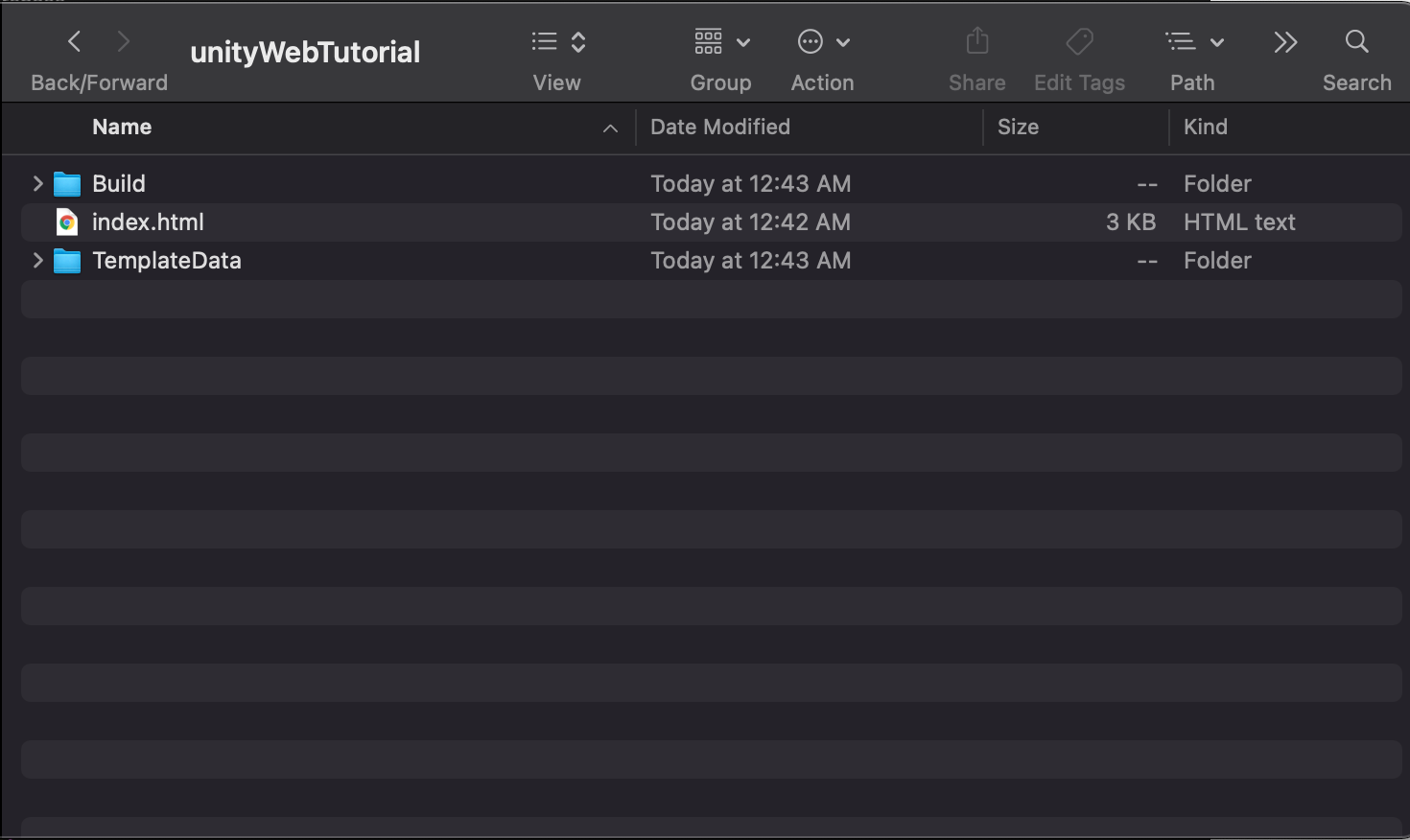
Open index.html in VS Code. Notice the unityInstance variable. We can use this to send a message back to Unity. Add this code under the full-screen button onClick listener. ( You can also comment out the code that makes the button full screen )
unityInstance.SendMessage('ExampleBridge', 'MessageFromJavascript', 'Hello from Javascript');
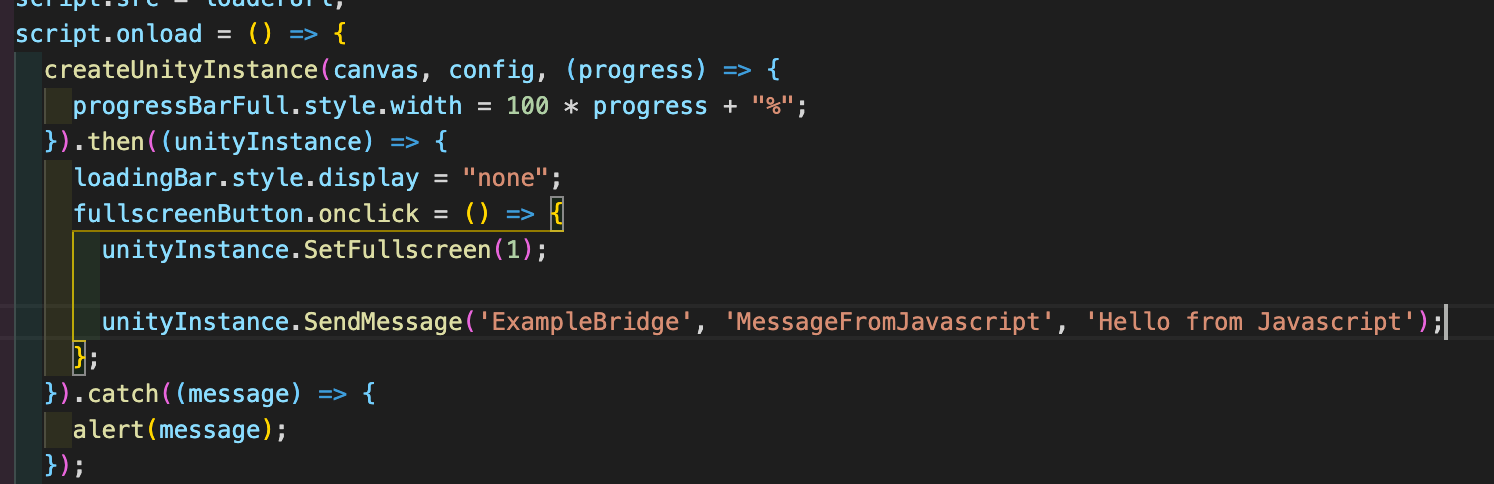
Let us walk through this code.
- The first parameter is the name of the GameObject we created, NOT the script.
- The second parameter is the name of the function in the script that is attached to our GameObject.
- The third parameter is the message we want to send.
You can run the built game now. You should get a popup as soon as the game loads, which is the message sent from Unity to Javascript.
Also when you click on the blue button with arrows ( the full-screen button ) you will see “Hello from Javascript in the browser’s console. This is the message from Javascript to Unity.
Here is also the Unity WebGL documentation for reference:
WebGL: Interacting with browser scripting
Thank you for taking the time to read this tutorial.