Flutter Flow: Get Value From Multiple-Choice Choice Chips
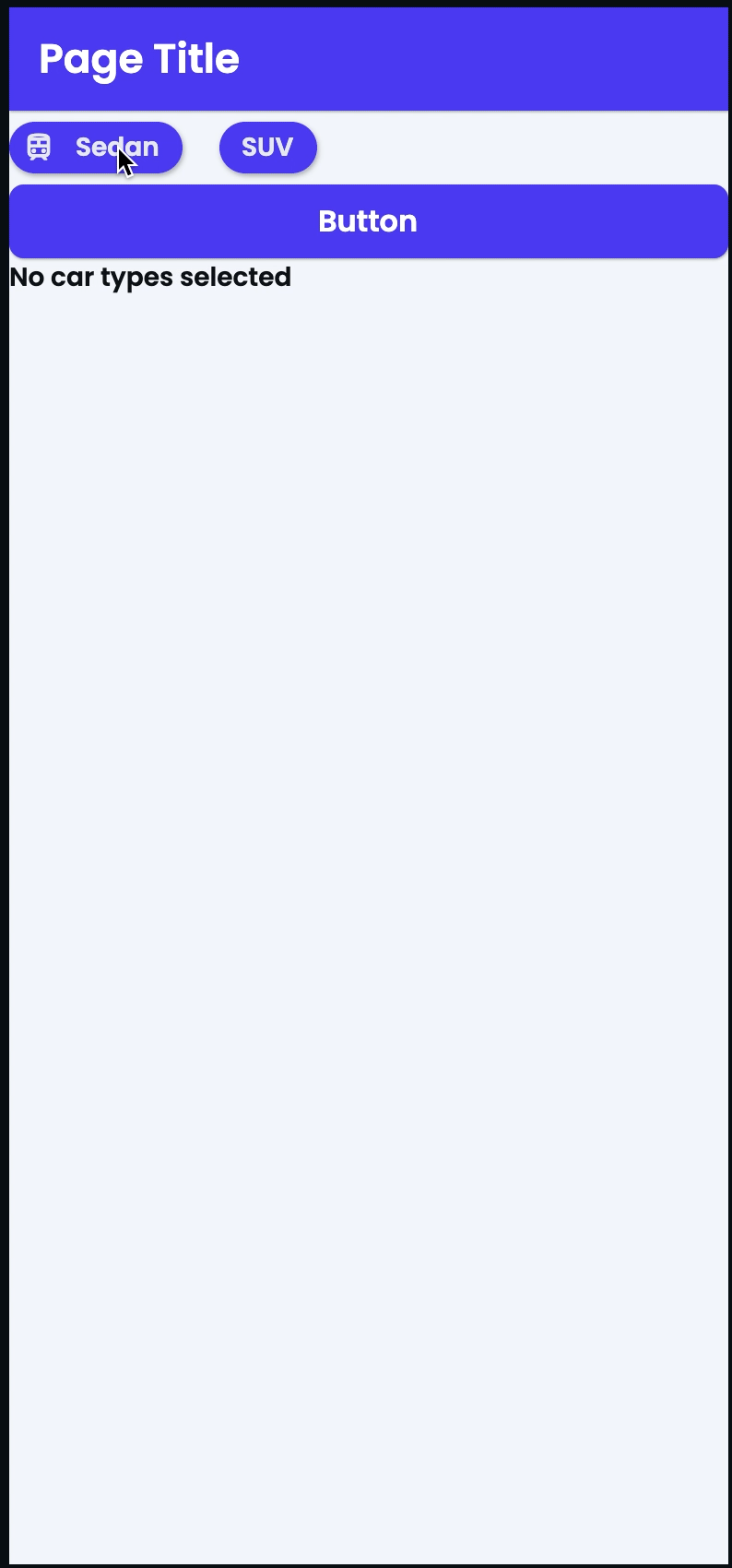
There is currently no built-in way in Flutter Flow to get the list of values from multiple-choice choice chips. I will show you an approach I took to solve this.
Custom development
I get a lot of requests for custom tweaks of Google Maps with Flutter.
I have created a form to capture these requests to better understand everyone’s needs. You can also request custom Flutter Flow development and/or Flutter Flow training.
https://coffeebytez.com/contact-me/
I will focus on the most highly requested items first, which will be available on my substack. I will also post exclusive in-depth tutorials there.
Please subscribe to my substack here:
https://coffeebytez.substack.com/?r=gdmkm&utm_campaign=pub-share-checklist
If you have urgent specific requests, please leave your contact information in the survey.
What are Choice Chips?
Choice Chips are material chips that let you choose multiple options. FlutterFlow’s implementation is a wrapper around Flutter’s choice chips with additional functionality.
ChoiceChips
ChoiceChip class – material library – Dart API
Example
I will walk through a small example to accomplish this. Start with a blank page and go to the UI Builder in FlutterFlow.
Search for choice chips in the UI Builder and drag it on your blank screen.
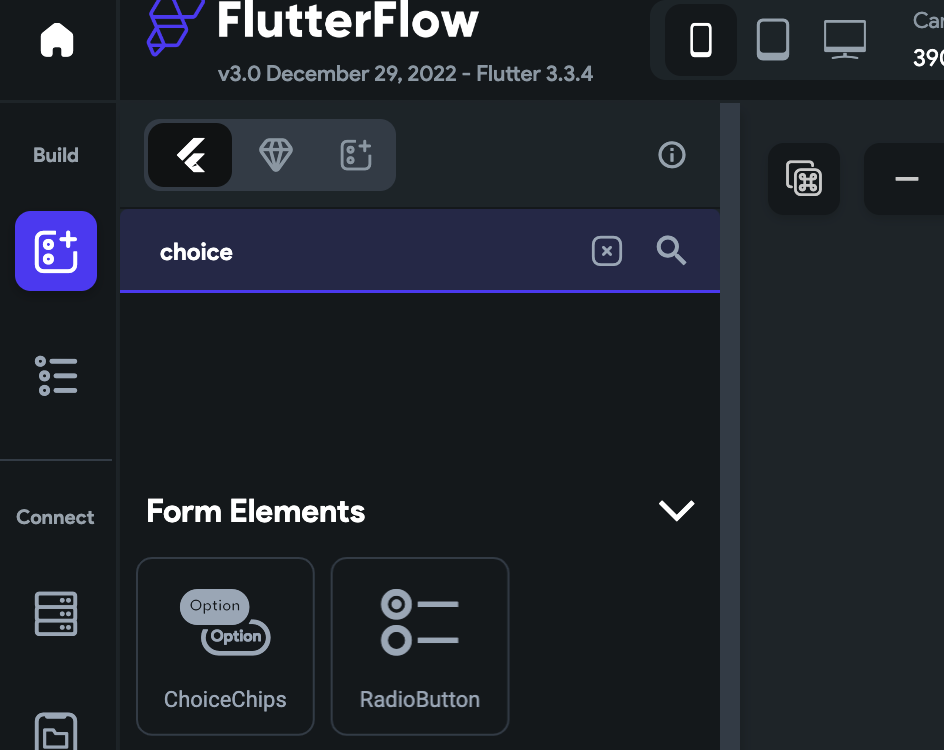
Add multiple options. For this example, I have two types of cars. Sedan and SUV. Also, turn the multiple-choice option on. Save the changes.
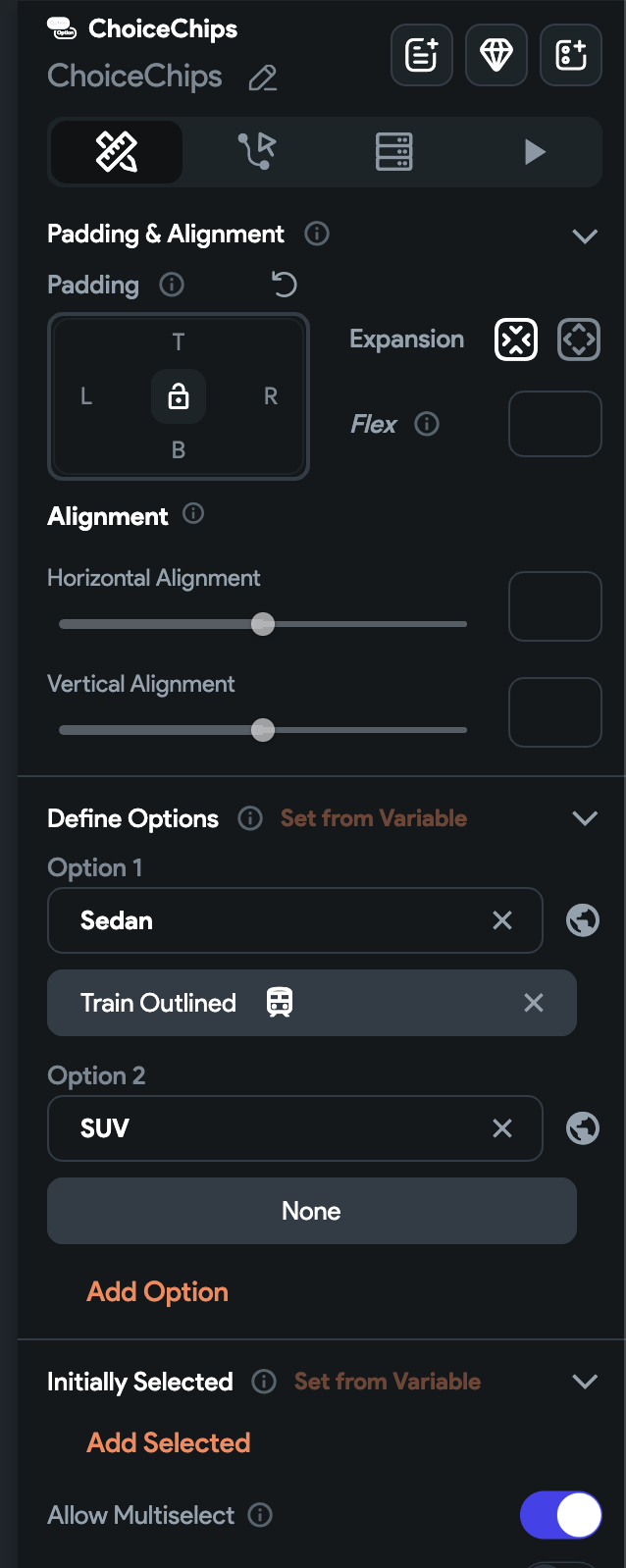
Drag a button and text field under the choice chips. Save the changes.
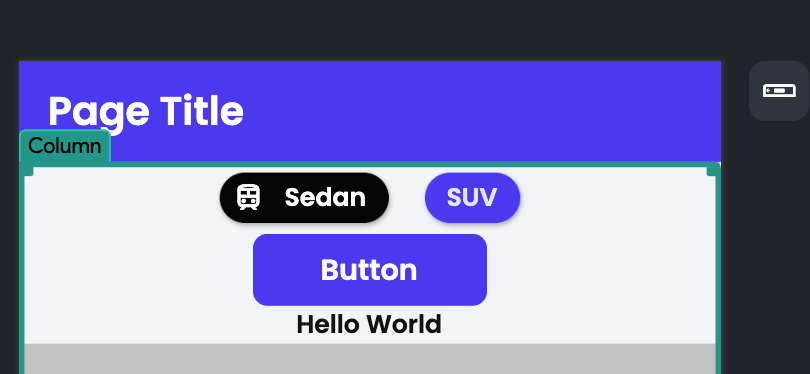
Note: Local State is now called App State in Flutter Flow
Now go to the Local State tab on the left-hand side of the screen. Create a local state of a list of strings. You may optionally choose to persist it.
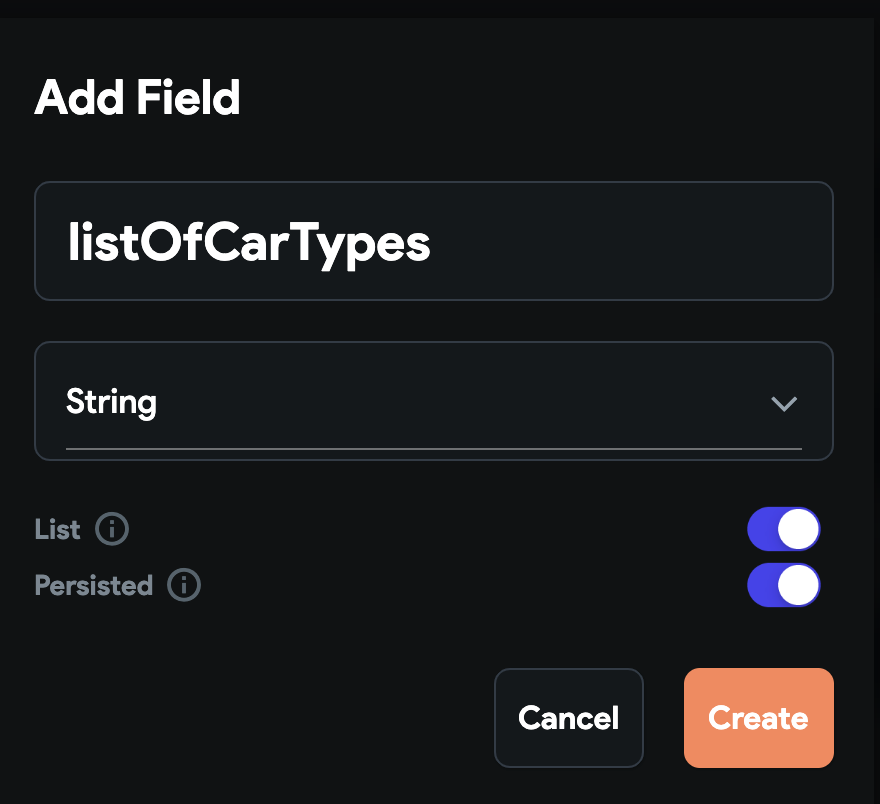

Also, create a string local state to save the single string of car types. Save the changes.

Go to the UI builder and set the text field to the local state string variable. Save the changes.
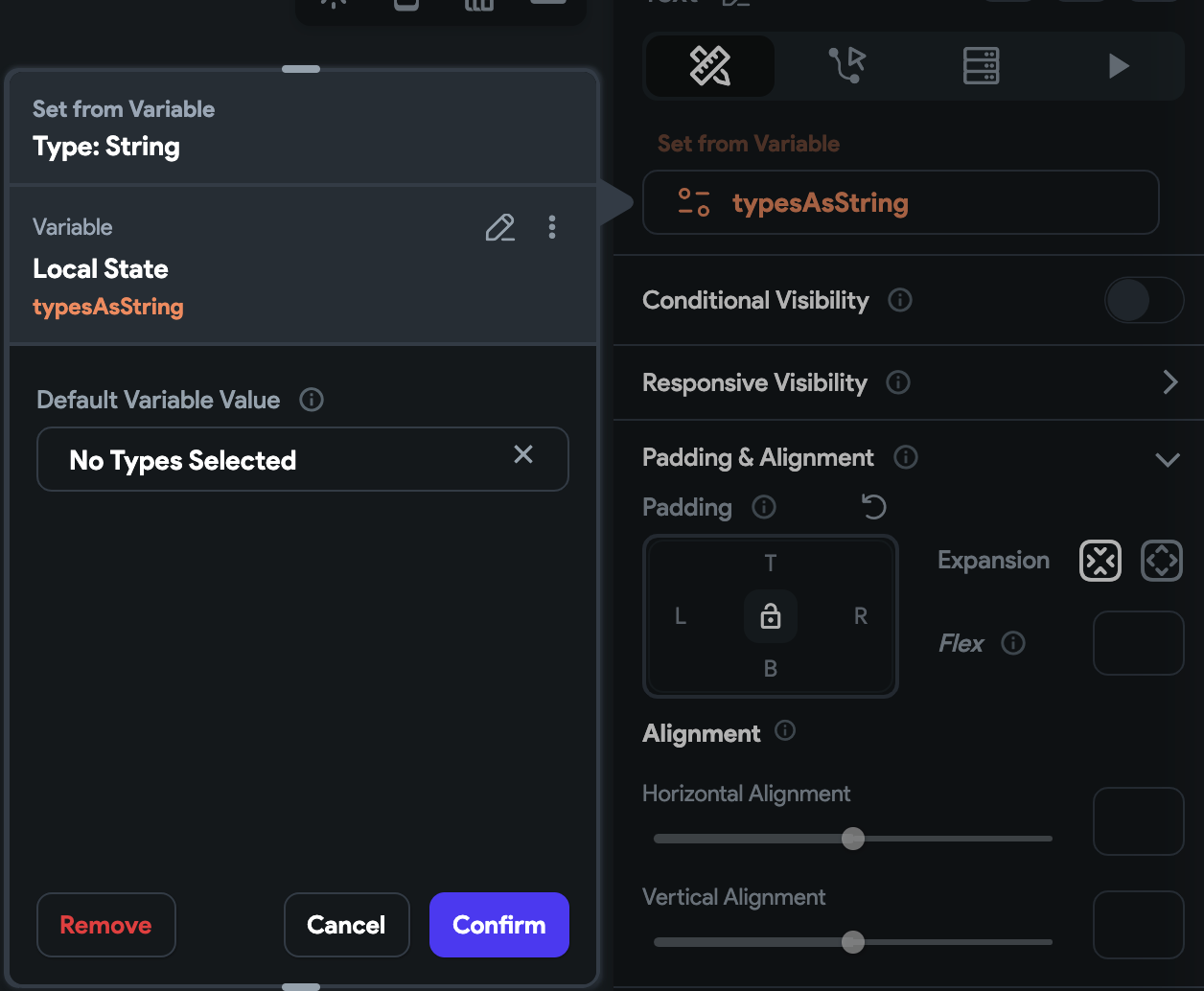
Click on the button so that we can create an action for it. Choose On Tap as the action.
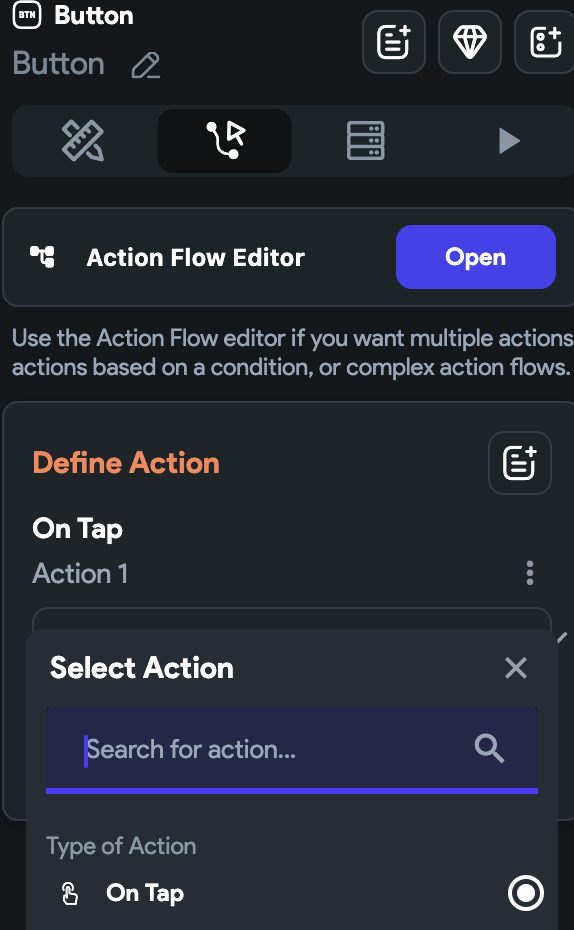
Scroll to the bottom to create a new action. You may also click on the Custom Code tab on the left to create an action.
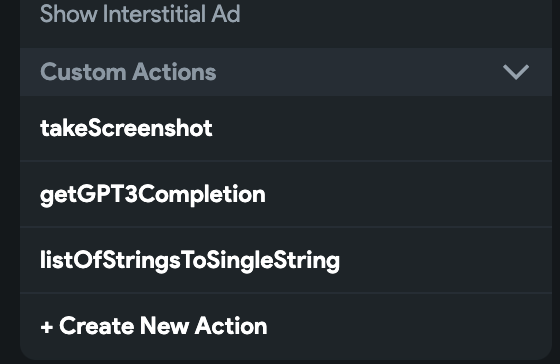
For the custom action, I downloaded and looked at the flutter flow source code to get an idea of how they handle updating state. For this custom action, we are creating an app state variable and using that to update the state. We are setting the single string state from the local string list state. Save and compile this custom action.
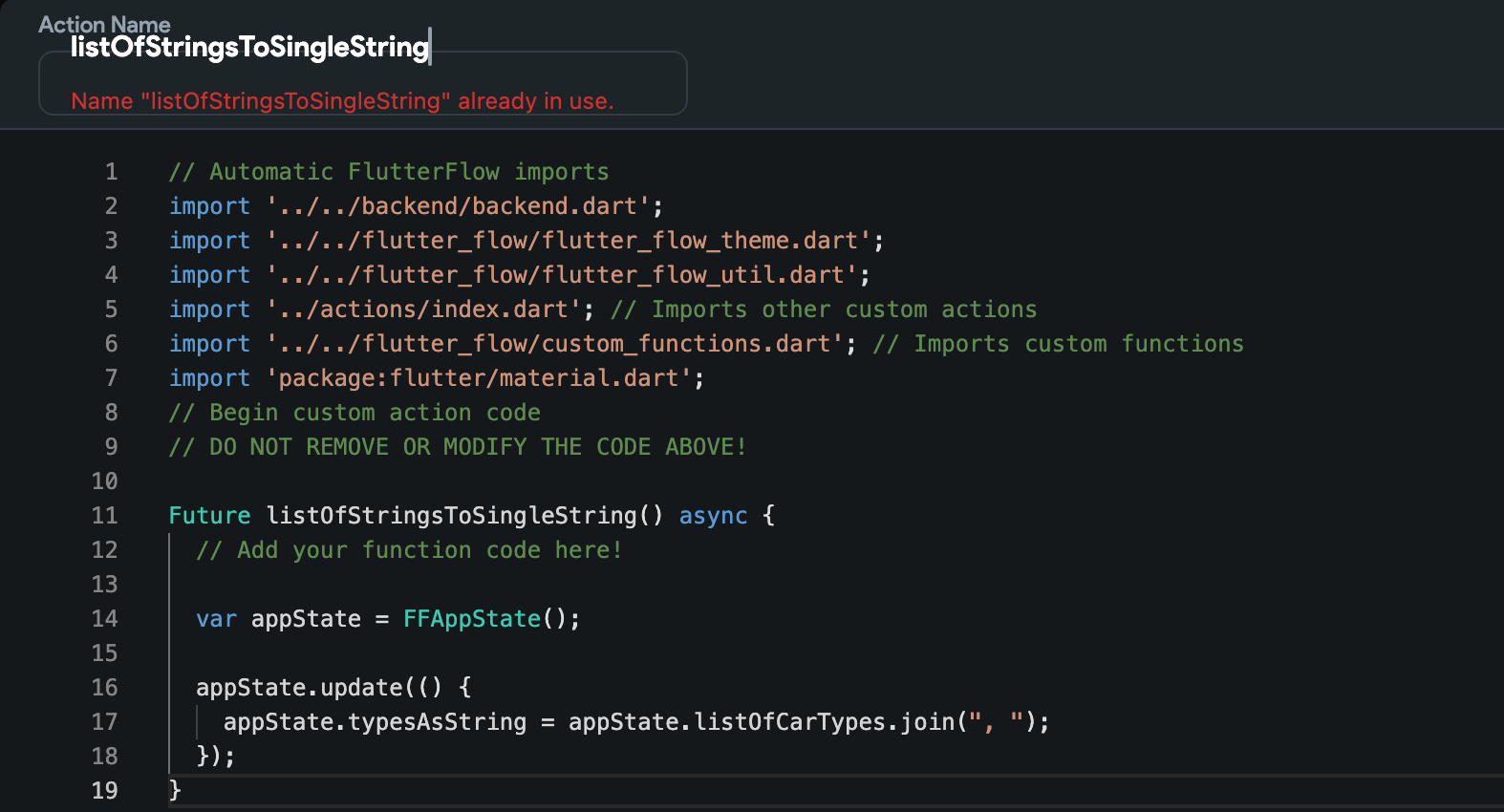
Now go back to the button and ensure the action is used when tapping it.
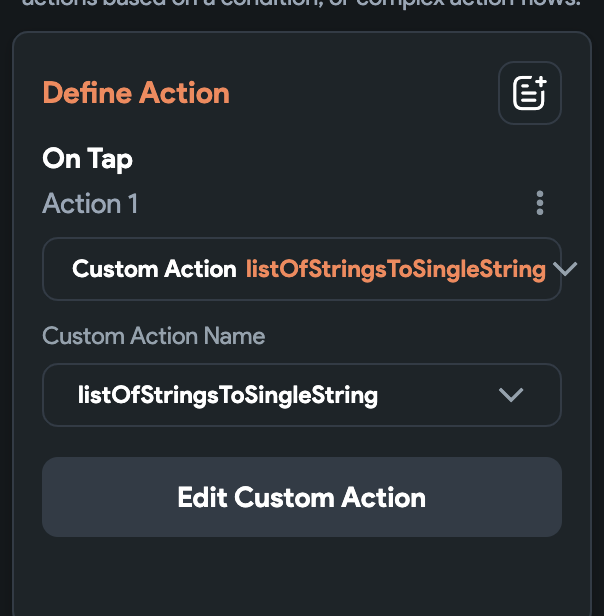
Click on the choice chips so that we can create an action for this. Choose On Selected and click Update Local State. For the Set Fields option, choose the list of strings local state. For the update type, choose Set Value. For the Value Source choose From Variable.
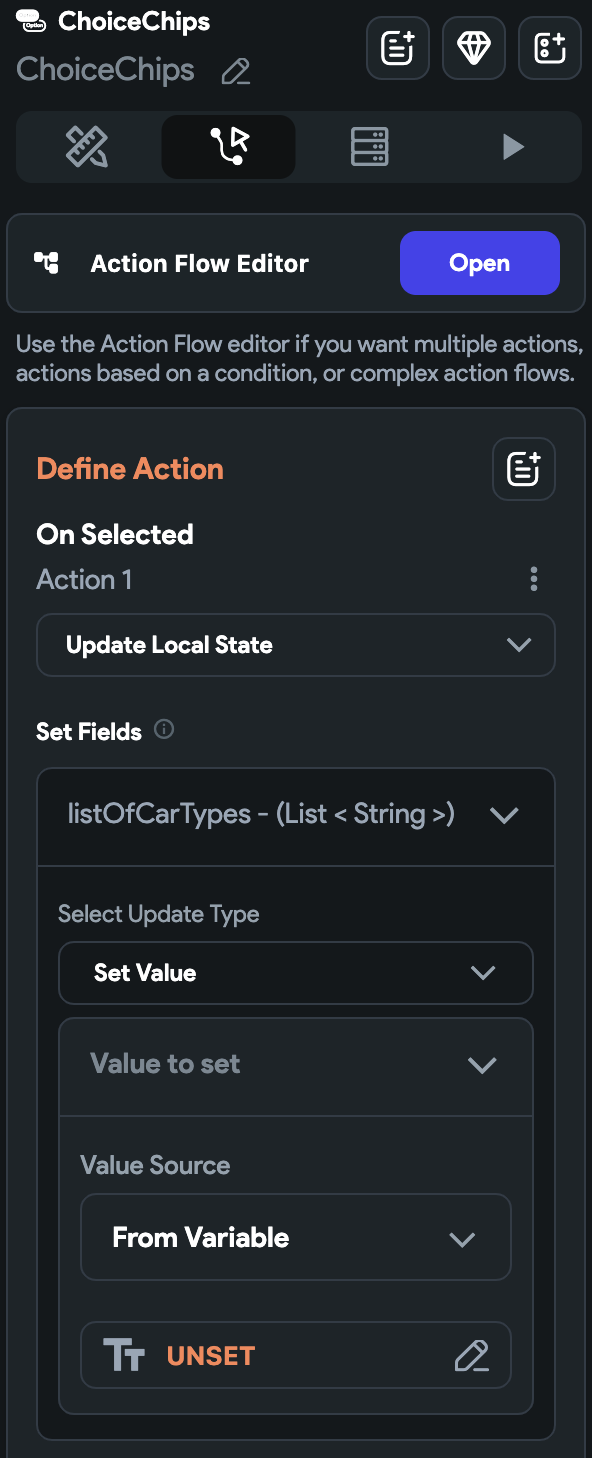
Now click the orange Unset text and choose Widget State. Now choose the Choice Chips. Save the changes.
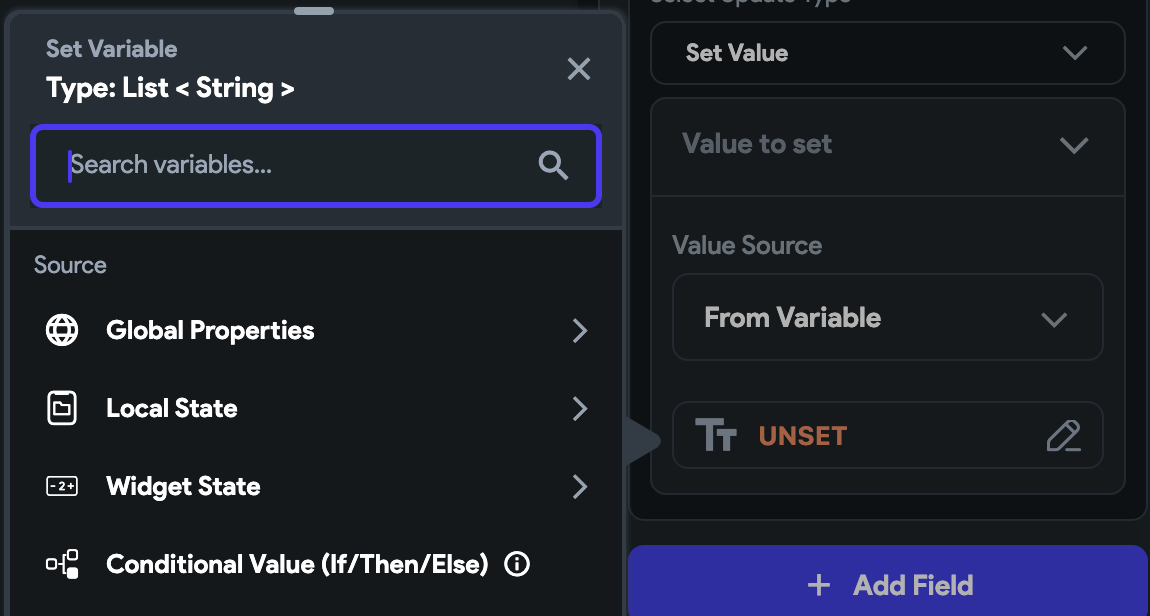
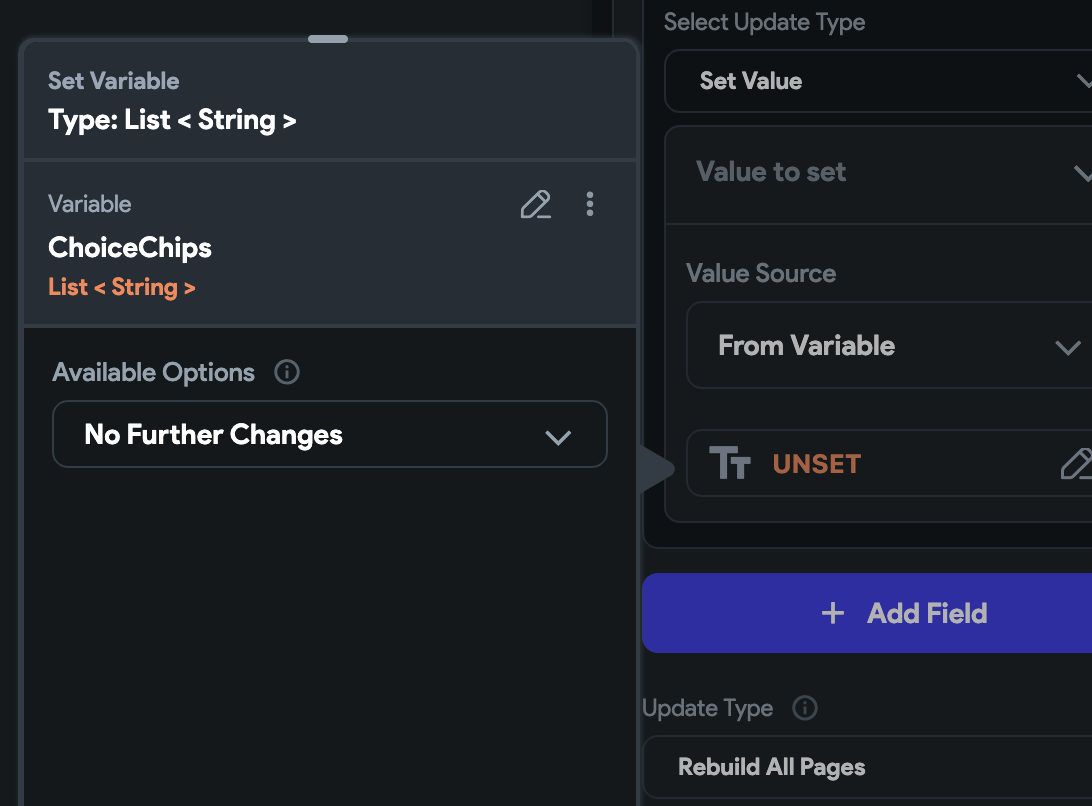
Now run the app, choose some choice chips, and click the button. The list of options should update the Text widget. This is a small example, but their are probably a lot more useful cases, such as making API requests using the local state values.
Source Code
// Automatic FlutterFlow imports
import '../../backend/backend.dart';
import '../../flutter_flow/flutter_flow_theme.dart';
import '../../flutter_flow/flutter_flow_util.dart';
import '../actions/index.dart'; // Imports other custom actions
import '../../flutter_flow/custom_functions.dart'; // Imports custom functions
import 'package:flutter/material.dart';
// Begin custom action code
// DO NOT REMOVE OR MODIFY THE CODE ABOVE!
Future listOfStringsToSingleString() async {
// Add your function code here!
var appState = FFAppState();
appState.update(() {
appState.typesAsString = appState.listOfCarTypes.join(", ");
});
}
Check out my other Flutter Flow articles
FlutterFlow Stepper Form with Firebase Firestore
Flutter Flow: Get Value From Multiple-Choice Choice Chips
Flutter Flow: Carousel Menu
Flutter Flow: Migrating the Carousel Menu to use data types
Flutter Flow: Adding Custom Markers for Google Maps
Flutter Flow: Use Supabase to show custom markers on Google Maps