Flutter Flow: Extracting Latitude and Longitude from LatLng
When dealing with Google Maps and Place Picker on Flutter Flow, there often arises a requirement to extract and store the latitude and longitude details.
These coordinates are essential for a variety of purposes, including populating your preferred database with separate columns for latitude and longitude. This process enables precise location tracking and management within your Flutter application.
Create a custom function
First, go to the Custom Code menu on the far left.
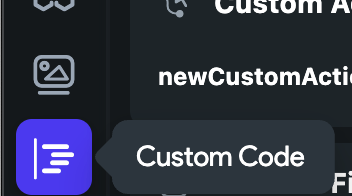
Update the function name to getCoordinate
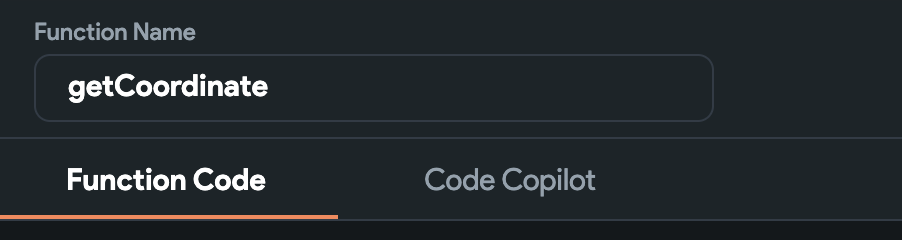
In the function settings set the return type to a Double. Now create two arguments, coordinates of type LatLng and isLatitude of type Boolean.
Custom Functions | FlutterFlow Docs
Implement the function
Our code is simple. If isLatitude is true, we will return the latitude from the LatLng coordinates object. If isLatitude is false we will return longitude.
if (isLatitude) {
return coordinates?.latitude ?? 0.0;
}
return coordinates?.longitude ?? 0.0;
Now click Save, then go over two buttons to the box with the exclamation mark and check and click that button. It validates our code.
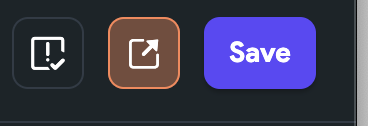
Here is the full code in case it helps. There are imports that Flutter Flow adds that we don’t need for our use case, but we can leave them alone for now.
import 'dart:convert';
import 'dart:math' as math;
import 'package:flutter/material.dart';
import 'package:google_fonts/google_fonts.dart';
import 'package:intl/intl.dart';
import 'package:timeago/timeago.dart' as timeago;
import '/flutter_flow/lat_lng.dart';
import '/flutter_flow/place.dart';
import '/flutter_flow/uploaded_file.dart';
import '/flutter_flow/custom_functions.dart';
import '/backend/schema/structs/index.dart';
import '/backend/supabase/supabase.dart';
import '/auth/supabase_auth/auth_util.dart';
double? getCoordinate(
LatLng? coordinates,
bool isLatitude,
) {
/// MODIFY CODE ONLY BELOW THIS LINE
if (isLatitude) {
return coordinates?.latitude ?? 0.0;
}
return coordinates?.longitude ?? 0.0;
/// MODIFY CODE ONLY ABOVE THIS LINE
}
Using our custom function to populate a field
For this example, we want to add latitude and longitude in their respective columns in Supabase. I have a parameter for a page that I’m passing a latLng variable to.
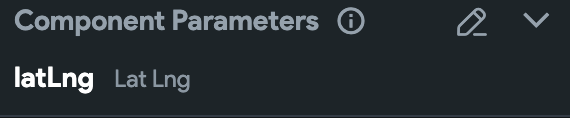
On the page that has this parameter, I have a button with an action to insert a row into my place table.
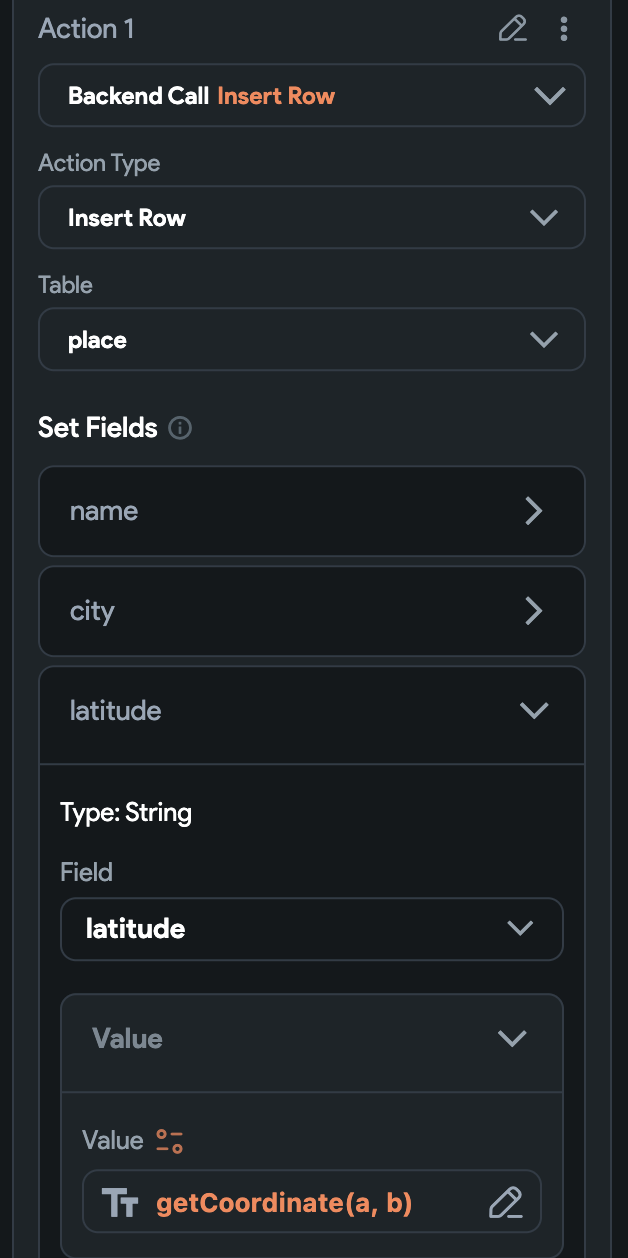
For the value of latitude select the orange, settings button to the right of the text Value. In the popup, find Custom Functions and choose our getCoordinate function.
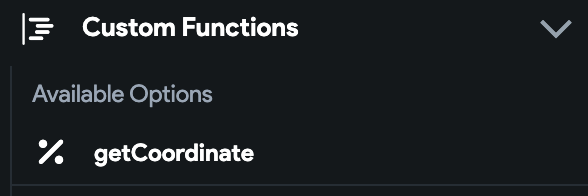
for the coordinates field, choose our latLng parameter and set our isLatitude param to True. For longitude follow the same steps, except for isLatitude you want to set it to False.

Click confirm then save your changes. If all goes well you will see your latitude and longitude in your database of choice.

I have created a form to better understand everyone’s needs. You can also request custom Flutter Flow development and/or Flutter Flow training.
https://coffeebytez.com/contact-me/