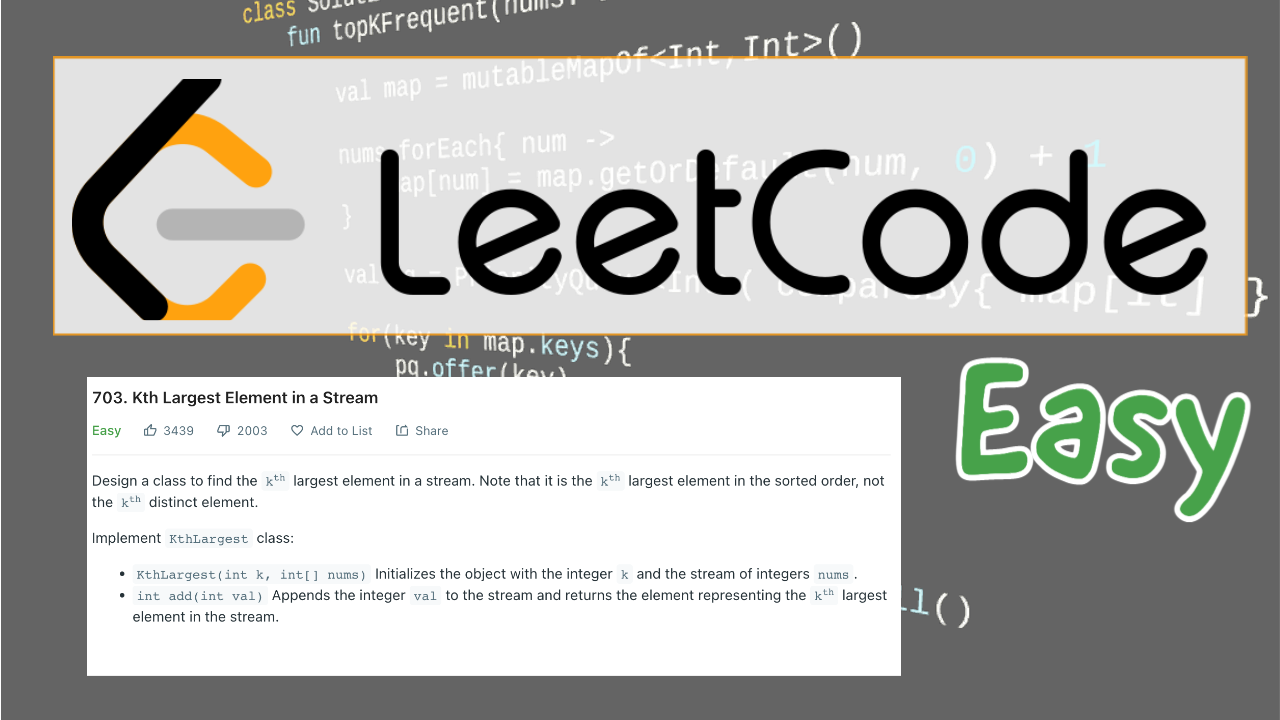
Leetcode: Kth Largest Element in a Stream (Kotlin)
Leetcode: Kth Largest Element in a Stream (Kotlin)
This is an easy question on leetcode. They give you an array and an integer k. They also give you an add function to complete. Kth Largest Element in a Stream - LeetCode
Problem Statement
Examples
Constraints
Understanding The Problem
There is an array of integers provided and an integer k. They also give you an add function to complete. The add function takes an integer and must be inserted into the array.
You must sort the array and return the kth largest element. The explanation in the example does a good job of walking through this.
Since our array must be sorted in ascending order, our k element should be k elements from the end.
Brainstorm
First, we need variables to hold the data provided by the constructor. We could use an array list, to make it easier to insert data anywhere in the array. This will especially come in handy in the add function. We can initially add everything to the array, then sort it using .sorted() on the array list. We can do the same steps in the add function.
I also thought of using a min-heap priority queue. I worked on a medium Leetcode problem not too long ago and thought I could take a similar approach to solving the problem. Leetcode: Top K Frequent Elements (Kotlin)
We will just add everything to the priority queue. Every time we add something to the priority queue, we will need to check if the priority queue’s size is bigger than k. If it is bigger, we will remove the value at the front by calling poll(). This will ensure we always have k number of elements so we can use peek() to find the kth largest element.
Solution
class KthLargest(k: Int, nums: IntArray) {
private val pq = PriorityQueue<Int>()
private val kth = k
init{
for(num in nums){
pq.offer(num)
if(pq.size > k) pq.poll()
}
}
fun add(`val`: Int): Int {
pq.offer(`val`)
if(pq.size > kth) pq.poll()
return pq.peek()
}
}
/**
* Your KthLargest object will be instantiated and called as such:
* var obj = KthLargest(k, nums)
* var param_1 = obj.add(`val`)
*/