Migrating away from Firebase, to Netlify and Supabase
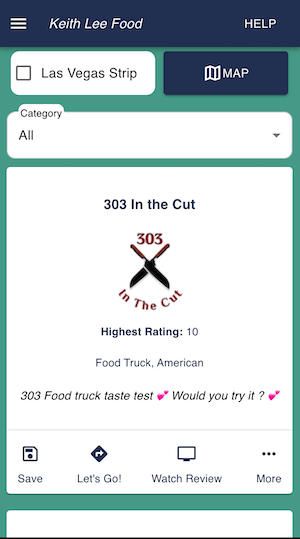
In my previous article, I showed you how to create a Keith Lee food app using ReactJS, ChatGPT, and Firebase.
However, as time passed, I found that Supabase for the backend and Netlify for hosting are more cost-efficient solutions.
Supabase is also open-source; you don’t have to deal with vendor lock-in as you would with Firebase. This means I can migrate to any hosting provider freely if I want to.
You also don’t have to host and maintain the entire Supabase project. You can connect to any database as a service platform.
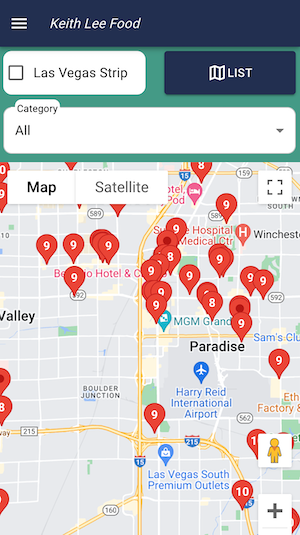
As you can tell from the screenshot, I have added more features, such as authentication, saving places, a new UI, filters, a guided tour, and a new “profile” page for each place.
Due to the complexity of the new features, I have also utilized redux-toolkit. I will write separate articles on my approaches to implementing these new features.
I also have created native iOS and Android apps using SwiftUI and Jetpack Compose. I will go over their implementation in the future as well.
Setting Up Supabase
Supabase is an open-source alternative to Firebase that offers different services, including database, authentication, storage, and real-time subscriptions. To start using Supabase, you need to create an account and a new project.
- Head over to https://supabase.com/ and sign up for a free account.
- Create a new project and fill in the required details.
Once your project is created, you’ll receive a unique project URL and API key. Save these for later use.
Migrating Data from Firestore to Supabase
Before migrating data from Firestore to Supabase, we need to export data from Firestore.
Supabase has a Firebase migration tool. I’ll list the relevant steps from the documentation so you don’t have to jump between tabs.
Firestore Data Migration
Exporting Firebase data
First, we must clone the firebase-to-supabase repo
git clone https://github.com/supabase-community/firebase-to-supabase.git
In the /firestore directory, create a file named supabase-service.json with the following contents:
{
"host": "database.server.com",
"password": "secretpassword",
"user": "postgres",
"database": "postgres",
"port": 5432
}
- Go to the Database settings for your project in the Supabase Dashboard.
- Under Connection Info, copy the Host string and replace the entry in your supabase-service.json file.
- Enter the password you used when you created your Supabase project in the password entry in the supabase-service.json file.
Generate a Firebase private key
- Log in to your Firebase Console and open your project.
- Click the gear icon next to Project Overview in the sidebar and select Project Settings.
- Click Service Accounts and select Firebase Admin SDK.
- Click Generate new private key.
- Rename the downloaded file to firebase-service.json.
Now we need to export the data to json. Run the following command with your collection name.
node firestore2json.js <collection-name>
In my case, the command will look like the following:
node firestore2json.js places
Import the JSON file into Supabase using the following command
node json2supabase.js <path_to_json_file>
Updating React App to Use Supabase
Now, we need to update our React app to use Supabase instead of Firestore. First, install the Supabase JavaScript client:
npm install @supabase/supabase-js
Also, check out the Supabase Quick Start documentation for React:
Use Supabase with React
The Supabase Javascript docs mention creating the following file to initialize the Supabase client. I’ve slightly changed it to allow using React environment variables.
import {createClient} from "@supabase/supabase-js";
export const supabase = createClient(
process.env.REACT_APP_SUPABASE_URL,
process.env.REACT_APP_SUPABASE_KEY,
)
// supabaseClient.js
Now create a file called .env at the root of your project. Each env variable must begin with the prefix REACT_APP_
Add the following to the .env file. Note you can find these under Settings > API.
REACT_APP_SUPABASE_URL=<your-supabase-url>
REACT_APP_SUPABASE_KEY=<your-supabase-key>
You can read more about React env vars in their official documentation:
Adding Custom Environment Variables | Create React App
Now let’s update our code to retrieve the data from Supabase.
useEffect(() => {
getPlaces
}, []);
const getPlaces = async() =>{
const { data, error } = await supabase.from("places").select().order('title');
setData(data);
}
Migrating from Firebase Hosting to Netlify
Using Netlify is easier to set up than Firebase.
Head over to https://www.netlify.com/ and create a new account. I signed up using Github.
After signing up, Netlify guides you on creating a new project. After creating your project, you can deploy it.
First, before deploying your project, you will need to add a _redirects file to the project directory of your React project.
/* /index.html 200
Check out the Netlify documentation for more configuration options:
Redirects and rewrites
The easiest way to deploy it depends on your experience.
You can allow Netlify to read your projects from Github, build them, and deploy them.
A Step-by-Step Guide: Deploying on Netlify
You can also create a production build and upload the build folder directly.
npm run build --production
Check out the latest updates to the Keith Lee food app:
Keith Lee Food App
Thank you for taking the time to read this article!